Exercise 4.03
The aim of this exercise is to play a game of Battleships using variables and
functions to keep track of your score and number of shots taken.
Files Needed
Click here to download the file needed for this exercise.
Instructions
- Extract and open the Battleships Game.xlsm
workbook. You'll find a worksheet containing a version of
the Wise Owl Battleships game.
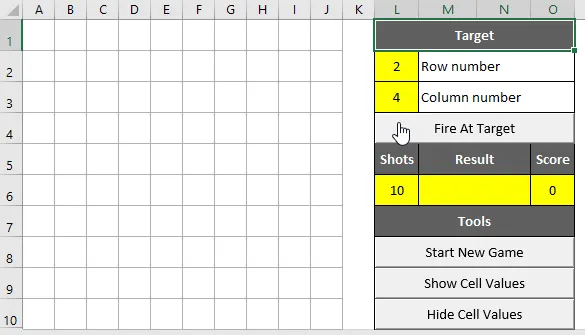
Clicking the
Fire At Target button should check whether the cell at the specified row and column contains a value and take the appropriate action.
- Create a function called Target_Is_OK
which returns a Boolean value. This
function should return True when the user has
entered a valid row and column number. It should return
False if any of the following conditions are
met:
- Cell L2 or L3 is empty.
- The value in cell L2 or L3
is not a number.
- The value in cell L2 or L3
is not between 1 and 10.
- In the Fire_At_Target subroutine,
check if the result of the Target_Is_OK
function is False. If so, place a message
in cell M6 and exit from the subroutine:
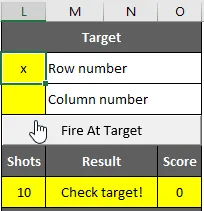
You could try changing the font or background colour of cell
M6 to make the message stand out.
- In the Fire_At_Target subroutine
declare a Range variable called
TargetCell and set it to
reference the cell at the specified row and column number.
Make sure that you do this after the code which checks that the
target is valid.
- Create a new subroutine called Colour_Target_Cell
with a single parameter which accepts a reference to a
Range object. Add code to this subroutine which
checks the value of the Range object passed to
it - if the cell is empty, colour the cell in grey, otherwise
colour it in red.
- In the Fire_At_Target subroutine, add a
call to the Colour_Target_Cell subroutine and
pass the TargetCell variable to it.
- Create a new subroutine called Set_Result_Cell
with a single parameter which accepts a String
value. Add code to this subroutine which sets the value of
cell M6 according to the value of the
String parameter:
Parameter value |
Result message |
Empty string |
Miss! |
A |
Hit Aircraft Carrier! |
B |
Hit Battleship! |
C |
Hit Cruiser! |
D |
Hit Destroyer! |
S |
Hit Submarine! |
- In the Fire_At_Target subroutine,
add a call to the Set_Result_Cell subroutine
and pass the value of the TargetCell variable
to it.
- Create a new function called Target_Score
which returns and Integer and has a single
parameter which accepts a String. Set the
return value of the function according the value of the
String parameter:
Parameter value |
Return value |
Empty string |
0 |
A |
4 |
B |
5 |
C |
3 |
D |
2 |
S |
1 |
- Declare a module-level variable called
GameScore which can hold an Integer
value. In the Fire_At_Target subroutine,
add an instruction which sets the value of the GameScore
variable to equal itself plus the result of the
Target_Score function (you'll need to pass the value of
the TargetCell variable to the function in
order to do this). Add another instruction to set the
value of cell O6 to be equal to the value of
the GameScore variable.
- In the Start_New_Game subroutine, add an
instruction which sets the value of the GameScore
variable to 0. Add another instruction
which sets the value of cell O6 to be equal to
the value of the GameScore variable.
- Declare a module-level variable called
ShotsRemaining which can hold an Integer
value.
- In the Start_New_Game subroutine, add an
instruction which sets the ShotsRemaining
variable to 10. Add another instruction
which sets the value of cell L6 to equal the
value of the ShotsRemaining variable.
- At the start of the Fire_At_Target
subroutine, check if the value of the ShotsRemaining
variable is 0 or less. If so, display a
message in cell M6 which reads "Game
Over!" and exit from the subroutine.
- At the end of the Fire_At_Target
subroutine, add an instruction which subtracts 1
from the value of the ShotsRemaining variable.
Add another instruction which sets the value of cell L6
to equal the value of the ShotsRemaining
variable.
- Return to the worksheet and click the Start New Game
button. Try playing a few rounds of the game and check
that everything works as expected.
- Save and close the workbook.
Answer Files
Click here to download a file containing a suggested answer.